A visual respresentation of which numbers are divisible by others, as a means of gaining intuition/finding patterns.
read more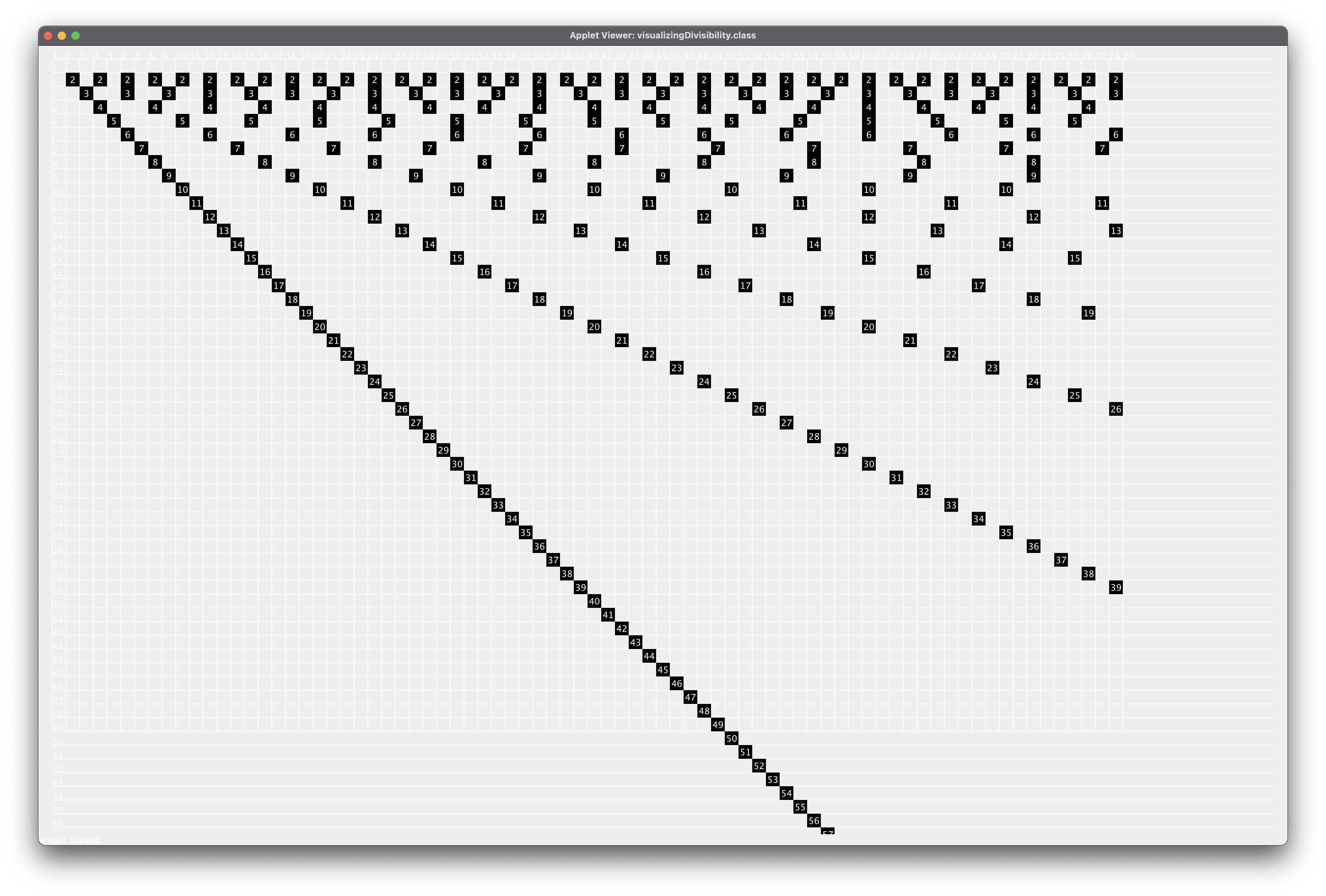
import java.awt.*;
import java.applet.*;
import java.io.FileNotFoundException;
public class visualizingDivisibility extends Applet {
/*
Applet will paint special shapes and use colors and fonts
Only new methods are explained
*/
// Specify variables that will be needed everywhere, anytime here
// The font variable
Font bigFont;
// The colors you will use
Color redColor;
Color weirdColor;
Color bgColor;
int numberToGoUpTo = 80;
int sizeOfBoxes = 20;
public void init()
{
this.setSize(1820, 1150);
// Here we will define the varibles further
// Will use Arial as type, 16 as size and bold as style
// Italic and Plain are also available
bigFont = new Font("Arial",Font.BOLD,16);
// Standard colors can be named like this
redColor = Color.black;
// lesser known colors can be made with R(ed)G(reen)B(lue).
weirdColor = new Color(60,60,122);
bgColor = Color.black;
// this will set the backgroundcolor of the applet
}
public void stop()
{
}
// now lets draw things on screen
public void paint(Graphics g)
{
for(int i=1;i<numberToGoUpTo;i++){
g.drawString(Integer.toString(i), sizeOfBoxes*i, sizeOfBoxes);
g.drawLine(sizeOfBoxes*i, sizeOfBoxes, sizeOfBoxes*i, 1000);
}
for(int i=1;i<numberToGoUpTo;i++){
// g.drawString(Integer.toString(i), 20*i, 20);
g.drawLine(sizeOfBoxes, sizeOfBoxes*i, 1800, sizeOfBoxes*i);
}
for(int i=1;i<numberToGoUpTo;i++){
g.drawString(Integer.toString(i), sizeOfBoxes, (sizeOfBoxes*i)+sizeOfBoxes);
}
for(int i=1; i<numberToGoUpTo; i++){
for(int a=1; a<numberToGoUpTo; a++){
if(i%a==0&&a!=1){
g.setColor(Color.black);
g.fillRect(sizeOfBoxes*i, sizeOfBoxes*a, sizeOfBoxes, sizeOfBoxes);
g.setColor(Color.white);
if(Integer.toString(a).length()>1){
g.drawString(Integer.toString(a), (sizeOfBoxes*i)+2, (sizeOfBoxes*a)+15);
}else{
g.drawString(Integer.toString(a), (sizeOfBoxes*i)+6, (sizeOfBoxes*a)+15);
}
}
}
}
}
}